ASM I/O: Mastering Non-Blocking Techniques
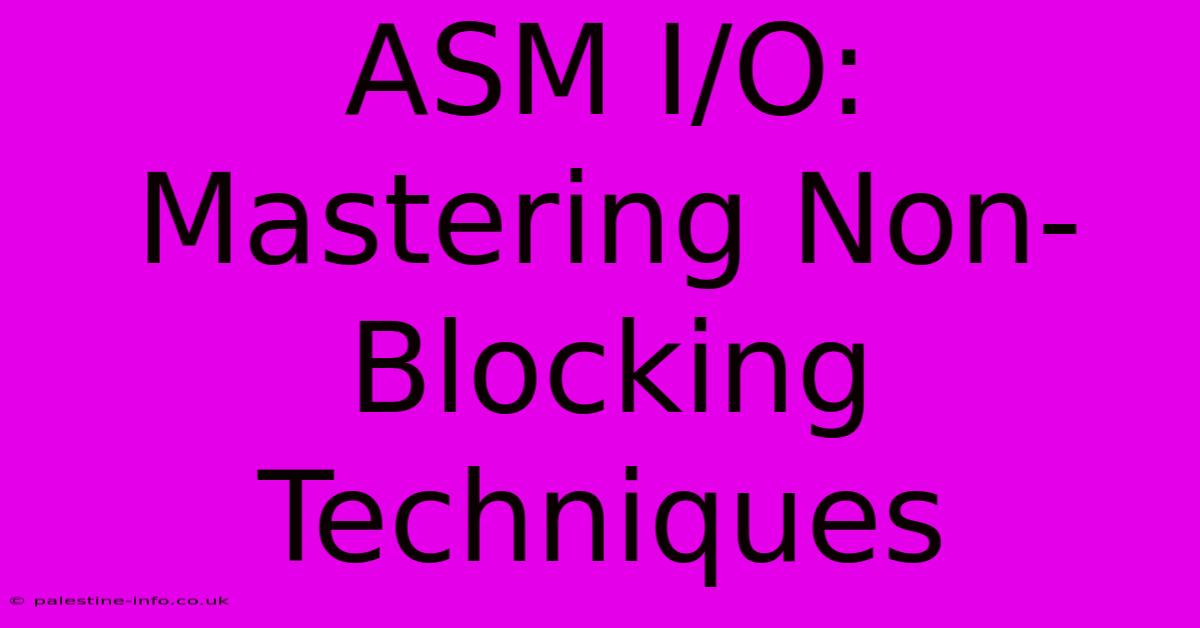
Table of Contents
ASM I/O: Mastering Non-Blocking Techniques
Asynchronous Input/Output (ASM I/O) is a crucial technique for building high-performance, responsive applications. Unlike traditional blocking I/O, which halts execution until an I/O operation completes, ASM I/O allows your program to continue executing other tasks while waiting for I/O operations to finish. This article delves into the intricacies of ASM I/O, exploring its benefits and demonstrating how to master its non-blocking techniques.
Understanding Blocking vs. Non-Blocking I/O
Before diving into ASM I/O, let's clarify the difference between blocking and non-blocking I/O.
Blocking I/O: When a program performs a blocking I/O operation (like reading from a file or network socket), it essentially pauses until the operation completes. This can lead to significant delays and reduced responsiveness, especially when dealing with slow I/O operations or network latency. Imagine a single-threaded application: if it's waiting for a network request, it cannot do anything else until that request is fulfilled.
Non-Blocking I/O: With non-blocking I/O, the program initiates an I/O operation and then continues execution without waiting for its completion. The program periodically checks the status of the operation or uses mechanisms like callbacks or events to handle the completion. This allows for concurrent execution and improved responsiveness.
The Advantages of ASM I/O
ASM I/O offers numerous advantages over traditional blocking I/O:
- Improved Responsiveness: Applications remain responsive even during lengthy I/O operations, enhancing the user experience.
- Increased Throughput: By overlapping I/O operations with other tasks, ASM I/O boosts overall throughput and efficiency.
- Better Resource Utilization: The system's resources are utilized more effectively, as the CPU isn't idly waiting for I/O operations to finish.
- Enhanced Scalability: ASM I/O is crucial for building scalable applications that can handle numerous concurrent requests.
Implementing Non-Blocking Techniques in ASM I/O
Implementing non-blocking techniques in ASM I/O depends heavily on the operating system and programming language you're using. Common strategies include:
1. Polling:
Polling involves periodically checking the status of an I/O operation. This is the simplest approach, but it can be inefficient if the I/O operation takes a long time to complete, as it wastes CPU cycles.
2. Asynchronous Events and Callbacks:
More sophisticated approaches leverage asynchronous events and callbacks. When an I/O operation completes, an event is triggered, and a registered callback function is executed to handle the result. This is a more efficient method than polling, as it avoids unnecessary CPU usage.
3. Select/Poll System Calls (Unix-like systems):
On Unix-like systems, system calls like select
and poll
allow you to monitor multiple I/O descriptors for readiness. This enables efficient management of multiple I/O operations concurrently.
4. epoll (Linux):
epoll
is a highly efficient mechanism for managing a large number of I/O events on Linux systems. It's significantly more performant than select
or poll
when dealing with many open sockets or files.
5. IOCP (Windows):
Windows provides the I/O Completion Ports (IOCP) mechanism, which offers an efficient way to handle asynchronous I/O operations. IOCP is highly scalable and well-suited for high-performance applications.
Example (Conceptual): Illustrating Non-Blocking Socket I/O
The following code snippet illustrates a conceptual example of non-blocking socket I/O. The specific implementation will vary depending on the operating system and programming language.
// Conceptual example - not production-ready code
// ... Socket setup ...
// Set socket to non-blocking mode
// ...
while (true) {
// Try to receive data
int bytesReceived = recv(socket, buffer, bufferSize, 0);
if (bytesReceived > 0) {
// Data received - process it
// ...
} else if (bytesReceived == 0) {
// Connection closed
// ...
} else if (errno == EWOULDBLOCK) {
// No data available yet - continue to other tasks
// ...
} else {
// Error occurred
// ...
}
// Perform other tasks
// ...
}
Note: This is a simplified illustration. Error handling and other crucial aspects are omitted for brevity. Real-world implementations require more robust error handling and might utilize more advanced techniques like asynchronous events or completion ports.
Conclusion
ASM I/O and its non-blocking techniques are essential for crafting responsive, high-performance applications. Mastering these techniques will significantly enhance your ability to build scalable and efficient software systems. Choosing the right approach—polling, callbacks, select
, epoll
, IOCP, or other methods—depends heavily on the specific requirements of your application and the operating system environment. Understanding the trade-offs between these different methods is critical for building optimal I/O solutions.
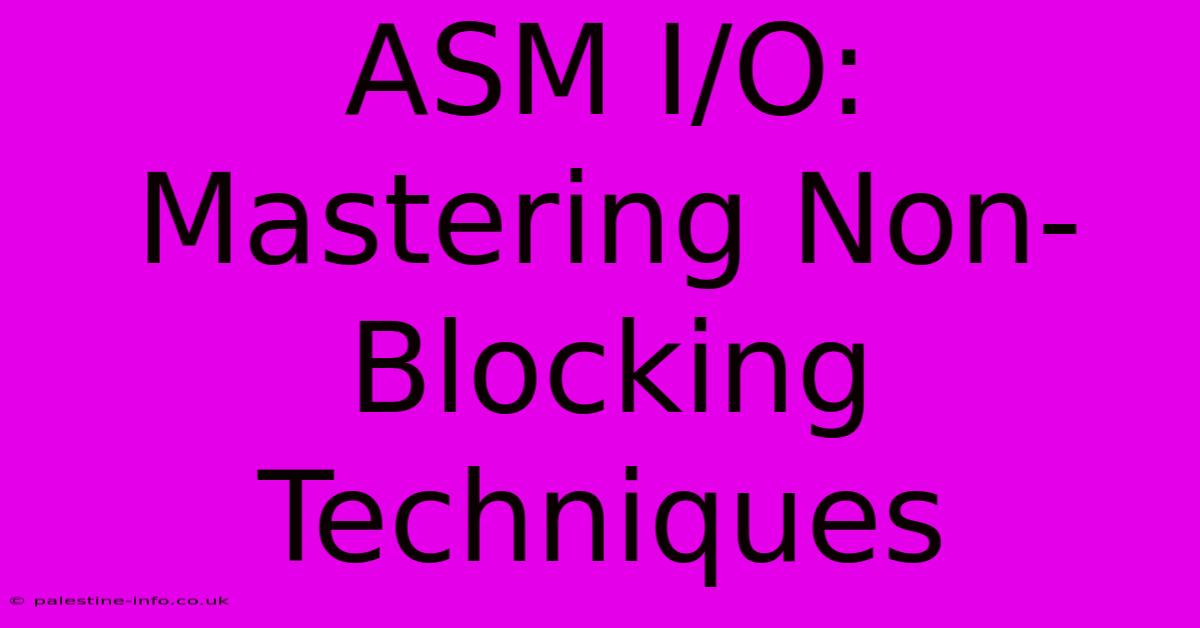
Thank you for visiting our website wich cover about ASM I/O: Mastering Non-Blocking Techniques. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Featured Posts
-
Eco Boost Mustang Long Term Reliability Report
Mar 16, 2025
-
Hanged Man Reversed A Deeper Understanding Of Self
Mar 16, 2025
-
Seraphina Hotel Barcelonas Artistic Hub
Mar 16, 2025
-
Dave Marcis Net Worth Revealed
Mar 16, 2025
-
Rouses Weekly Ad Houma Fresh Offers This Week
Mar 16, 2025